What is BLoC?
BLoC stands for Business Logic Components; it aims to separate the application’s business logic from User Interface, making the application code more unambiguous, scalable, and testable.
Flutter bloc is one of the state management for Flutter applications. Bloc allow you to separate the logic from the UI. Writing code in Bloc makes it easier to write and reuse tests.
Example: class DemoBloc extends Bloc<DemoEvent, DemoState> {
GraphBloc(this._graphRepositoty) : super(GraphLoadingState()) {}}
The DemoBloc class is a bridge between our UI and the Data class, In other words, this class will handle all the Events triggered by the User and sends the relevant State back to the UI.
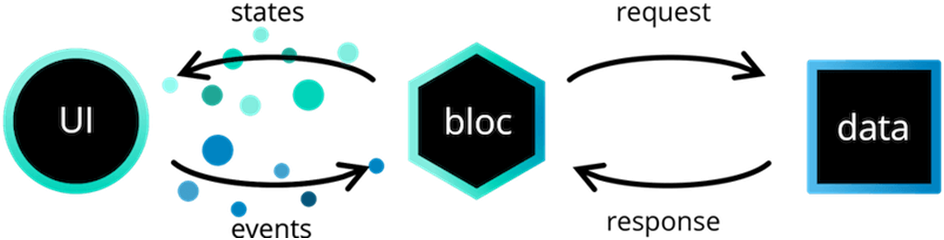
To understand it more, let’s say we want to fetch data from some kind of service. Then the UI will trigger the event TodoFetched
to the Bloc. The Bloc will initially have a state called TodoInitial
and then when we fetch the data from the repository, we can update the state to TodoLoaded
. Also, when we update the states, we also update the UI. We will see this in the example later on.
Adding Bloc to Flutter
First, you need to add the following dependency to the pubspec.yaml
file:
dependencies:
bloc: ^8.0.2
cupertino_icons: ^1.0.2
equatable: ^2.0.3
flutter:
sdk: flutter
flutter_bloc: ^8.0.1
http: ^0.13.4
dev_dependencies:
flutter_lints: ^1.0.0
flutter_test:
Click CTRL + S to save, and you have successfully added the above dependencies to your Flutter application!
The bloc
dependency is used because we also used cubit
in the example. The flutter_bloc
will provide you with the widgets necessary to make it easier to use the Bloc pattern in Flutter. The equatable
package is used to easily compare objects in Dart.
Observing Bloc Changes
In the following application, we would fetch data from the following url:
First, in the main.dart
file delete all the code and add the following:
When comparing objects, you would have to override both the ==
and the hashcode
method, so with equatable
you wouldn’t have to worry about that since it’s easily done with just one line of code.
The http
dependency is used to create http request to fetch, delete, update, create.
import ‘package:bloc/bloc.dart’;
import ‘package:flutter/material.dart’;
import ‘app.dart’;
import ‘todo_bloc_observer.dart’;
void main() async {
BlocOverrides.runZoned(
() => runApp(const App()),
blocObserver: TodoBlocObserver(),
);
}
The BlocOverrides
class contains the property blocObserver
which will enable us to observe any change in the Bloc and this would make it easier when getting stuck on some issue. Now create a file called todo_bloc_observer.dart
and add the following:
To understand how bloc works, we need to know what are events and states.
Event: events are an application’s inputs (like button press to load images, submit etc.).
Example:
abstract class DemoEvent {}
class Demo extends DemoEvent {}
2. State: States are simply the application’s state, which can be changed in response to the event received.The UI will update according to the State it receives from the Bloc. For example, there could be different kinds of states –
LoadingState – Will Show Progress Indicator
LoadedState – Will Show Actual widget with data
ErrorState – Will show an error that something went wrong.
Example: abstract class WeatherState {}
class DemoInitial extends DemoState {}
class DemoLoadInprogress extends DemoState {}
class DemoLoadSuccess extends DemoState {
final Demo Demo;
WeatherLoadSuccess({required this.Demo});
}
class DemoLoadFailure extends DemoState {
final String error;
DemoLoadFailure({required this.error});
}
Bloc manages these events and states, i.e., it takes a stream of Events and transforms them into a stream of States as output.
How Does its's Work?
When you use flutter bloc you are going to create events to trigger the interactions with the app and then the bloc in charge is going to emit the requested data with a state.
Bloc Widget:
BlocProvider: BlocProvider is used as dependency widget so that a single instance of a bloc can be provided to multiple widgets within a subtree. BlocProvider is put it at the place from where all the children can access the bloc.
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return BlocProvider(
create: (context) => //Bloc Class name,
child: MaterialApp(
home: //Home page ,
),
);
}
}
BlocBuilder: BlocBuilder is a widget that helps Re-building the UI based on State changes. It takes two things bloc, and state.
body: BlocBuilder<event,State>(
builder: (context, state) {
return …
}
)
Conclusion
We can conclude that the Flutter app proves itself as a useful application developer. It assists a lot in the field of programming and technology. Flutter app works better than the application of iOS and Android. The programmers, as well as non-programmers, can make such apps.
Dart provides the lowest run rate, and all the developing processes precede in a stable condition. Moreover, it can manipulate all the applications that need advancements for a more immeasurable version. We hope this article or an unbiased review of the Flutter app will be helpful for you.
